State management simplified with zustand
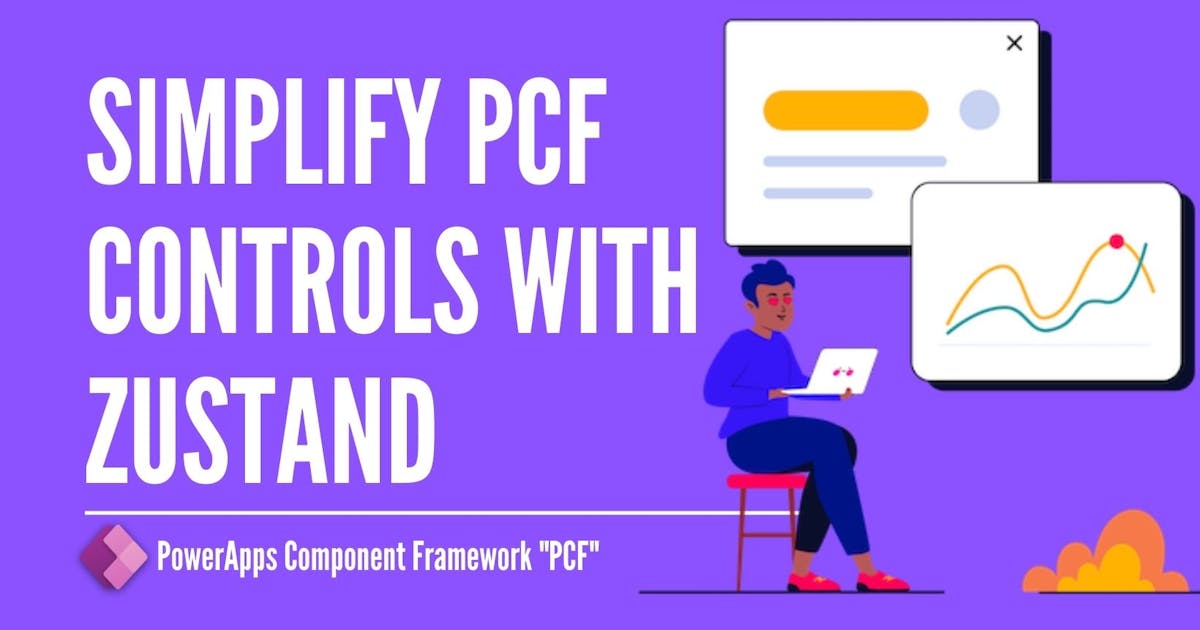
zustand is a small, fast and scalable state management package that you can use with your react applications, it can be used as your data store within your PCF control.
Below are the benefits of using it,
- Bundle size is 1.17 kb!
- Avoid props inheritance between your components
- Simplify the functions implementations for maintaining data across the control.
- Provides hooks to use the data store which handles re-renders
- Store can be called outside functional components
In our case in index.ts to get data in the getOutputs method
Check how to use it below,
Creating your data store
After initiating your control, create your store
//if you're using typescript, it would be better to set the type of the store
type PcfState= {
vars: any[]; //data object
add: (newVar: any) => void; //add function
set: (vars: any[]) => void; //set function
update: (_var: any) => void; //update function
remove: (schema: number) => void; //remove function
clear: () => void; //clear function
};
// the store below, holds the data objects and the functions related
const usePcfStore = create<PcfState>((set, get) => ({
vars: [],
//get().vars returns the current state of vars array
//set() sets the state of the store properties
add: (newVar: any) => set({ vars: [...get().vars, newVar] }),
set: (vals: any) => set({ vars: vals }),
update: (_var: any) =>
set({
vars: get().vars.map((_obj: any) => {
if (_obj.id === _var.id) {
_obj = _var;
}
return _obj;
}),
}),
remove: (id: number) =>
set({
vars: get().vars.filter((x) => x.id !== id),
}),
clear: () => set({ vars: [] }),
}));
export default useVarsStore;
Use the store hook in your component
const { add, set, vars, remove, update } = usePcfStore();
You can easily use the data store functions just like that
set(newVars);
and you get the data object like that
let _obj = vars; //just like that
Get the store data in the getOutputs method
//easy as this!
public getOutputs(): IOutputs {
return {
bindProperty : usePcfStore.getState().vars
};
}
Hope this makes it easier for you in your PCF development.
Published on:
Learn more